Hi, I have an issue over here.
I’m doing the part of changing the state of coin’s price but throw me this error on console.
( Warning: Can’t perform a React state update on an unmounted component. This is a no-op, but it indicates a memory leak in your application. To fix, cancel all subscriptions and asynchronous tasks in the componentWillUnmount method. )
This is my code.
import React, { Component } from 'react';
import './Coin.css';
import PropTypes from 'prop-types';
export default class Coin extends Component {
constructor(props){
super(props);
this.state = {
price: this.props.price
}
}
componentDidMount(){
const callback = () => {
const randomPercentage = 0.995 + Math.random() * 0.01;
this.setState( function(oldState) {
return{
price: oldState.price * randomPercentage
}
});
}
setInterval(callback, 1000);
}
render() {
return (
<tr className='coin-row'>
<td>{this.props.name}</td>
<td>{this.props.ticker}</td>
<td>${this.props.price}</td>
<td><button>Refresh</button></td>
</tr>
)
}
}
Coin.propTypes = {
name: PropTypes.string.isRequired,
ticker: PropTypes.string.isRequired,
price: PropTypes.number.isRequired
}
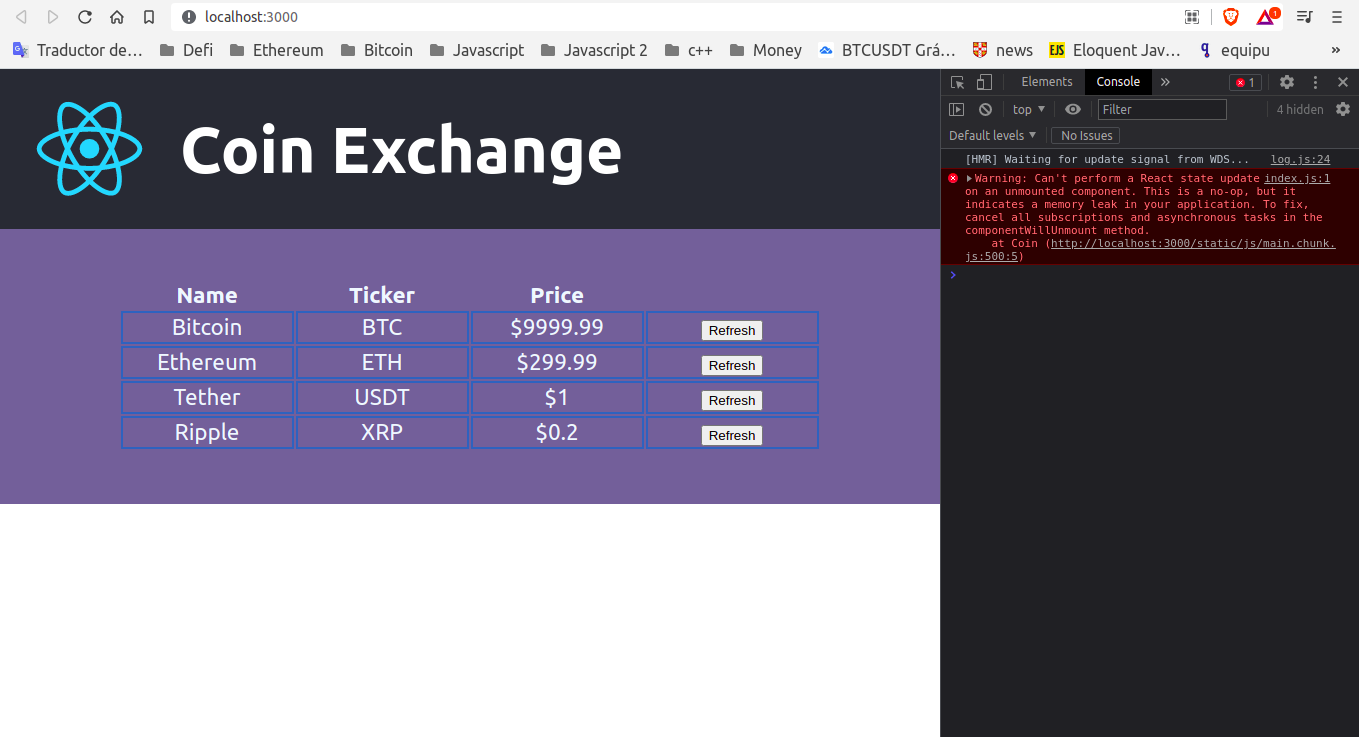