Hi @kenn.eth
Sorry for my late reply, because i moved ahead to finish the Ethereum Smart Contract Security Course and it was great learning and now I am revisiting this subject.
You have two clear errors.
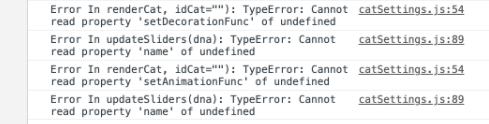
Well Below I am sharing with you my catSettings.js, catFactory.js, catConstants.js and colors.js code. I honestly have tried again but I couldn’t find any error ( or TypeError)
catSettings.js 
function getDna(){
try {
let dna = ''
dna += $('#dnabody').html()
dna += $('#dnamouth').html()
dna += $('#dnaeyes').html()
dna += $('#dnaears').html()
dna += $('#dnashape').html()
dna += $('#dnadecoration').html()
dna += $('#dnadecorationMid').html()
dna += $('#dnadecorationSides').html()
dna += $('#dnaanimation').html()
dna += $('#dnaspecial').html()
if (dna.length !== 16 ) throw `DNA string ('${dna}') length should be 16 (not ${dna.length} digits)`
return BigInt(dna)
}
catch (error) {
console.log(`Error In getDna(): ${error}`)
}
}
function renderCat(cat, idCat=""){
try {
headColor(cat.dna.headColor, idCat)
mouthColor(cat.dna.mouthColor, idCat)
eyesColor(cat.dna.eyesColor, idCat)
earsColor(cat.dna.earsColor, idCat)
eyeVariation(cat.dna.eyesShape, idCat)
decorationVariation(cat.dna.decorationPattern, idCat)
midDecorationColor(cat.dna.decorationMidColor, idCat)
sidesDecorationColor(cat.dna.decorationSidesColor, idCat)
animationStyle(cat.dna.animation, idCat)
// Display Special DNA digit
$(`${idCat} #dnaspecial`).html(cat.dna.lastNum) // Update DNA display (below cat)
// Display Cats' Generation
$(`${idCat}`).find('#catGenNum').html(cat.gen)
// Display Cats' Price (if it has one)
if (cat.price) $(`${idCat}`).find('#catPrice').html("PRICE: " + cat.price + " ETH")
// Display Cats' status
// $(`${idCat}`).find('#catStatus').html("TEST")
}
catch (error){
console.log(`Error In renderCat, idCat=""): ${error}`)
}
}
function updateSliders(dna){
try {
$('#bodycolor').val(dna.headColor) //Update slider's value
$('#headcode').html('code: '+dna.headColor) //Update slider's badge
$('#mouthcolor').val(dna.mouthColor)
$('#mouthcode').html('code: '+dna.mouthColor)
$('#eyescolor').val(dna.eyesColor)
$('#eyescode').html('code: '+dna.eyesColor)
$('#earscolor').val(dna.earsColor)
$('#earscode').html('code: '+dna.earsColor)
$('#eyesshape').val(dna.eyesShape)
$('#eyename').html(eyeVariations[dna.eyesShape].name)
$('#decorationpattern').val(dna.decorationPattern)
$('#decorationname').html(decorationVariations[dna.decorationPattern].name)
$('#decorationmidcolor').val(dna.decorationMidColor)
$('#midbirthmarkcode').html('code: '+dna.decorationMidColor)
$('#decorationsidescolor').val(dna.decorationSidesColor)
$('#sidebirthmarkscode').html('code: '+dna.decorationSidesColor)
$('#animationstyle').val(dna.animation)
$('#animationcode').html(animationVariations[dna.animation].name)
}
catch (error){
console.log(`Error In updateSliders(dna): ${error}`)
}
}
//EVENT LISTENERS
// Changing cat's Body' colors
$('#bodycolor').change(()=>{
var colorVal = $('#bodycolor').val()
$('#headcode').html('code: '+ colorVal) //This updates text of the badge next to the slider
headColor(colorVal)
})
// Changing Cat's Mouth color
$('#mouth_tail_color').change(()=>{
var colorVal = $('#mouth_tail_color').val()
$('#mouthcode').html('code: ' + colorVal) // This updates text of the badge next to the slider
mouthColor(colorVal)
})
//Changing cat's eyes color
$('#eyescolor').change(()=>{
var colorVal = $('#eyescolor').val()
$('#eyescode').html('code: ' + colorVal) // This updates text of the badge next to the slider
eyesColor(colorVal)
})
//Changing cat's ears colors
$('#earscolor').change(()=>{
var colorVal = $('#earscolor').val()
$('#earscode').html('code: ' + colorVal) // This updates text of the badge next to the slider
earsColor(colorVal)
})
//Changing Cat eyes Shape
$('#eyesshape').change(()=>{
var shape = parseInt($('#eyesshape').val())
$('#eyename').html(eyeVariations[shape].name)
eyeVariation(shape)
})
//Changing Decoration pattern
$('#decorationpattern').change(()=>{
var pattern = parseInt($('#decorationpattern').val())
$('#decorationname').html(decorationVariations[pattern].name)
decorationVariation(pattern)
})
//Changing The Side & Mid Birthmarks colors
$('#decorationsidescolor').change(()=>{
var colorVal = $('#decorationsidescolor').val()
$('#sidebirthmarkscode').html('code: '+ colorVal) //This updates text of the badge next to the slider
sidesDecorationColor(colorVal)
})
$('#decorationmidcolor').change(()=>{
var colorVal = $('#decorationmidcolor').val()
$('#midbirthmarkcode').html('code: '+ colorVal) //This updates text of the badge next to the slider
midDecorationColor(colorVal)
})
//Changing the Animation Style :
$('#animationstyle').change(()=>{
var animationVal = parseInt($('#animationstyle').val())
$('#animationcode').html(animationVariations[animationVal].name)
animationStyle(animationVal)
})
catFactory.js 
//This function changes the color of Head and Chest
function headColor(code,idCat = "") {
$(`${idCat}.cat__head, ${idCat}.cat__chest`).css('background', '#' + colors[code]) //This changes the color of the cat
$(`${idCat}#dnabody`).html(code) //This updates the body color part of the DNA that is displayed below the cat
}
// This function changes the color of Mouth and tail
function mouthColor(code,idCat = ""){
$(`${idCat}.cat__mouth-left, ${idCat}.cat__mouth-right, ${idCat}.cat__tail`).css('background', '#' + colors[code]) //This changes the Cat's Mouth and Tail Colors
$(`${idCat}#dnamouth`).html(code) // This updates the Mouth and Tail color part of the DNA that is displayed below the cat
}
//This function changes the color of Cat's eyes
function eyesColor(code, idCat = ""){
$(`${idCat}.cat__eye span.pupil-left, ${idCat}.cat__eye span.pupil-right`).css('background', '#' + colors[code]) //This changes the Cat's Mouth and Tail Colors
$(`${idCat}#dnaeyes`).html(code) // This updates the Mouth and Tail color part of the DNA that is displayed below the cat
}
//This function changes the color of Cat's ears and Paws
function earsColor(code, idCat = ""){
$(`${idCat}.cat__ear--left, ${idCat}.cat__ear--right, ${idCat}.cat__paw-left, ${idCat}.cat__paw-right`).css('background', '#' + colors[code]) //This changes the Cat's ears Colors
$(`${idCat}#dnaears`).html(code) //This updates the Ears color part of the DNA that is displayed below the Cat
}
//###################################################
//USING BELOW FUNCTIONS TO CHANGE CATTRIBUTES
//###################################################
//Changing Eye shapes
function eyeVariation(num, idCat = "") {
normalEyes(idCat) //Reset eyes
$(`${idCat}#dnashape`).html(num)//This updates the Eyes shape part of the DNA that is displayed below the Cat
eyeVariations[num].setEyesFunc(idCat)
}
//########################################################################################
// switch (num) {
// case 1:
// normalEyes()
// $('#eyename').html('Basic Eyes')// Set the badge of the eye to 'Basic'
// break
// case 2:
// normalEyes()//reset
// $('#eyename').html('Chill Eyes')//Set the badge to "Chill"
// eyesType1()//Change the eye shape by bringing a Solid border-top 15 px
// break
// case 3:
// normalEyes()//reset
// $('#eyename').html('Smile Eyes')//Set the badge to "Smile"
// eyesType2()//Change the eye shape by bringing a Solid border- bottom15 px
// break
// case 4:
// normalEyes()//reset
// $('#eyename').html('Cool Eyes')//Set the badge to "Cool"
// eyesType3()//Change the eye shape by bringing a Solid border-right 15 px
// break
// case 5:
// normalEyes()//reset
// $('#eyename').html('Dotted Eyes')//Set the badge to "Dotted Eyes"
// eyesType4()//Change the eye shape by bringing a border-style: dotted
// break
// }
function normalEyes(idCat) {
//Reset eye Lids to fully open
$(`${idCat}.cat__eye`).find('span').css('border', 'none')
// Reset pupil to round and centered
$(`${idCat} .cat__eye`).find('span').css('width', '42px')
$(`${idCat} .pupil-left`).css('left', '42px')
$(`${idCat} .pupil-right`).css('left', '166px')
}
function eyesType1(idCat) {
$(`${idCat}.cat__eye`).find('span').css('border-top', '15px solid')
}
function eyesType2(idCat) {
$(`${idCat}.cat__eye`).find('span').css('border-bottom', '15px solid')
}
function eyesType3(idCat) {
$(`${idCat}.cat__eye`).find('span').css('border-right', '15px solid')
}
function eyesType4(idCat) {
$(`${idCat}.cat__eye`).find('span').css('border-style', 'Dotted')
}
//###################################################################################
//Changing Cat BirthMark Dimensions + Rotation
function decorationVariation(num, idCat="") {
normalDecoration(idCat)
$(`${idCat} #dnadecoration`).html(num) // Update DNA display (below cat)
decorationVariations[num].setDecorationFunc(idCat)
}
//#########################################################################################
// switch (num) {
// case 1:
// normalDecoration()
// $('#decorationname').html('Basic Forehead Patches')// Set the Badge to Decoration pattern Basic
// break
// case 2:
// normalDecoration()
// $('#decorationname').html('Angled Forehead Patches')// Set the Badge to Decoration pattern - Angled patches
// normalDecoration1()
// break
// case 3:
// normalDecoration()
// $('#decorationname').html('Smaller Forehead Width Patches')// Set the Badge to Decoration pattern - Smaller Patches
// normalDecoration2()
// break
// case 4:
// normalDecoration()
// $('#decorationname').html('Smaller Forehead Height Patches')// Set the Badge to Decoration pattern - Smaller Patches
// normalDecoration3()
// break
// }
function normalDecoration(idCat) {
//Remove all style from other decorations
//In this way we can also use normalDecoration() to reset the decoration style
$(`${idCat}.cat__head-dots`).css({ "transform": "rotate(0deg)", "height": "48px", "width": "14px", "top": "1px", "border-radius": "0 0 50% 50%" })
$(`${idCat}.cat__head-dots_first`).css({ "transform": "rotate(0deg)", "height": "35px", "width": "14px", "top": "1px", "border-radius": "50% 0 50% 50%" })
$(`${idCat}.cat__head-dots_second`).css({ "transform": "rotate(0deg)", "height": "35px", "width": "14px", "top": "1px", "border-radius": "0 50% 50% 50%" })
}
function normalDecoration1(idCat) {
//Remove all style from other decorations
//In this way we can also use normalDecoration() to reset the decoration style
$(`${idCat}.cat__head-dots`).css({ "transform": "rotate(0deg)", "height": "48px", "width": "14px", "top": "1px", "border-radius": "0 0 50% 50%" })
$(`${idCat}.cat__head-dots_first`).css({ "transform": "rotate(45deg)", "height": "35px", "width": "14px", "top": "1px", "border-radius": "50% 0 50% 50%" })
$(`${idCat}.cat__head-dots_second`).css({ "transform": "rotate(-45deg)", "height": "35px", "width": "14px", "top": "1px", "border-radius": "0 50% 50% 50%" })
}
function normalDecoration2(idCat) {
//Remove all style from other decorations
//In this way we can also use normalDecoration() to reset the decoration style
$(`${idCat}.cat__head-dots`).css({ "transform": "rotate(0deg)", "height": "48px", "width": "8px", "top": "1px", "border-radius": "0 0 50% 50%" })
$(`${idCat}.cat__head-dots_first`).css({ "transform": "rotate(0deg)", "height": "35px", "width": "8px", "top": "1px", "border-radius": "50% 0 50% 50%" })
$(`${idCat}.cat__head-dots_second`).css({ "transform": "rotate(0deg)", "height": "35px", "width": "8px", "top": "1px", "border-radius": "0 50% 50% 50%" })
}
function normalDecoration3(idCat) {
//Remove all style from other decorations
//In this way we can also use normalDecoration() to reset the decoration style
$(`${idCat}.cat__head-dots`).css({ "transform": "rotate(0deg)", "height": "24px", "width": "8px", "top": "1px", "border-radius": "0 0 50% 50%" })
$(`${idCat}.cat__head-dots_first`).css({ "transform": "rotate(0deg)", "height": "20px", "width": "8px", "top": "1px", "border-radius": "50% 0 50% 50%" })
$(`${idCat}.cat__head-dots_second`).css({ "transform": "rotate(0deg)", "height": "20px", "width": "8px", "top": "1px", "border-radius": "0 50% 50% 50%" })
}
//########################################################################################################
//This changes the Side BirthMark Colors
function sidesDecorationColor(code, idCat= "") {
$(`${idCat}.cat__head-dots_first , ${idCat}.cat__head-dots_second`).css('background', '#' + colors[code]) //This changes the color of the cat's Side BirthMark
$(`${idCat}#dnadecorationSides`).html(code) //This updates the Side Birthmarks color part of the DNA that is displayed below the cat
}
//This changes the Mid BirthMark colors
function midDecorationColor(code, idCat ="") {
$(`${idCat}.cat__head-dots`).css('background', '#' + colors[code]) //This changes the color of the cat's Mid Birthmark
$(`${idCat}#dnadecorationMid`).html(code) //This updates the Mid Birthmark color part of the DNA that is displayed below the cat
}
//#################################################################################################################
//This Function changes the animation style
function animationStyle(num,idCat = ""){
resetAnimation(idCat) //Reset animations
$(`${idCat} #dnaanimation`).html(num) // Update DNA display (below cat)
animationVariations[num].setAnimationFunc(idCat)
}
//#################################################################################################################
// switch (num) {
// case 1 :
// movingHeadAnimation()
// $('#animationcode').html('Moving Head')//It sets the Badge to Moving Head style
// break
// case 2 :
// bigHeadAnimation()
// $('#animationcode').html('Big Head') // It sets the Badge to Scaled Head style
// break
// case 3 :
// translatedHeadAnimation()
// $('#animationcode').html("Translated Head")//It setsthe Badge to Transitioned/Transported Head style
// break
// case 4 :
// movingTailAnimation()
// $('#animationcode').html('Moving Tail')// It sets the badge to Moving Tail animation style
// break
// }
function animationType1(idCat) { //Head rotates and moves
$(`${idCat}#head`).addClass('movingHead')
}
function animationType2(idCat) { //Head gets scaled
$(`${idCat}#head`).addClass('scalingHead')
}
function animationType3(idCat) { //Head gets translated
$(`${idCat}#head`).addClass('translatingHead')
}
function animationType4(idCat) { //Tail starts Moving
$(`${idCat}#tail`).addClass('movingTail')
}
function resetAnimation(idCat){
$(`${idCat}#head`).removeClass('movingHead')
$(`${idCat}#head`).removeClass('scalingHead')
$(`${idCat}#head`).removeClass('translatingHead')
$(`${idCat}#tail`).removeClass('movingTail')
}
catConstants.js 
var colors = Object.values(allColors())
const defaultDNA = {
"headColor" : 10,
"mouthColor" : 13,
"eyesColor" : 96,
"earsColor" : 10,
//Cattributes
"eyesShape" : 1,
"decorationPattern" : 1,
"decorationMidColor" : 13,
"decorationSidesColor" : 13,
"animation" : 0,
"lastNum" : 1
}
const defaultCat = {
id: "",
dna: defaultDNA,
gen: ""
}
const eyeVariations = [
{
"name" : "Basic Eyes",
"setEyesFunc" : normalEyes
},
{
"name" : "Smiling Eyes",
"setEyesFunc" : eyesType1
},
{
"name" : "Shy Girl eyes",
"setEyesFunc" : eyesType2
},
{
"name" : "Chill",
"setEyesFunc" : eyesType3
},
{
"name" : "Dotted Eyes",
"setEyesFunc" : eyesType4
}
]
const decorationVariations = [
{
"name" : "Standard Stripes",
"setDecorationFunc" : normalDecoration
},
{
"name" : "Angular Stripes",
"setDecorationFunc" : normalDecoration1
},
{
"name" : "Big Stripes",
"setDecorationFunc" : normalDecoration2
},
{
"name" : "Small Stripes",
"setDecorationFunc" : normalDecoration3
}
]
const animationVariations = [
{
"name" : "Moving Head",
"setAnimationFunc" : animationType1
},
{
"name" : "Scaling Head",
"setAnimationFunc" : animationType2
},
{
"name" : "Translating Head",
"setAnimationFunc" : animationType3
},
{
"name" : "Moving Tail",
"setAnimationFunc" : animationType4
}
]
colors.js 
function allColors(){
return colorPallete;
}
var colorPallete = {00: "ae494f",
01: "9a3031",
02: "8cd42e",
03: "a0417a",
04: "91c656",
05: "299f7a",
06: "c65d1e",
07: "b2bbd6",
08: "2d4024",
9: "4b5715",
10: "ffcc80",
11: "3f1174",
12: "b22a90",
13: "fff3e0",
14: "4c858b",
15: "18bebe",
16: "b5044b",
17: "d6b1d4",
18: "fecb40",
19: "748882",
20: "4a3c95",
21: "482916",
22: "267bf0",
23: "5af7e2",
24: "adeacc",
25: "cf2b03",
26: "b3c459",
27: "353f9",
28: "5d4993",
29: "ba8d15",
30: "da2457",
31: "ff17fe",
32: "d6e81d",
33: "daf2db",
34: "19b510",
35: "18e26f",
36: "b7c36a",
37: "8cb175",
38: "bdce32",
39: "f2e0ba",
40: "a2f8a5",
41: "64bf50",
42: "f1a771",
43: "4982a9",
44: "f66c41",
45: "2fe802",
46: "bda142",
47: "8342ff",
48: "2b4ab4",
49: "ad4595",
50: "bae4f",
51: "b76d01",
52: "8e8207",
53: "285b9f",
54: "c4422a",
55: "f1eaa7",
56: "e3a0cc",
57: "65c116",
58: "656ccf",
59: "7c25f4",
60: "1e18d1",
61: "688a7d",
62: "1fe786",
63: "425716",
64: "4ac043",
65: "547836",
66: "24a216",
67: "fd9bba",
68: "24894d",
69: "c54b03",
70: "6fbdce",
71: "cff1dd",
72: "8805fb",
73: "fe99d2",
74: "c52f14",
75: "e31c54",
76: "d010eb",
77: "b83436",
78: "c294b6",
79: "564a6c",
80: "531bcf",
81: "c04b8c",
82: "3cd2ef",
83: "82286c",
84: "aa2639",
85: "86be6c",
86: "e62102",
87: "5471fc",
88: "5c089",
89: "703c75",
90: "9a8e8f",
91: "8b9307",
92: "fcbc82",
93: "ea5978",
94: "b8e370",
95: "43474b",
96: "262d2b",
97: "ddd67e",
98: "344867"}
//Random color
function getColor() {
const randomColor = Math.floor(Math.random() * 16777215).toString(16);
return randomColor
}
//Generate colors for pallete
function genColors(){
const colors = []
for(const i = 10; i < 99; i ++){
const color = getColor()
colors[i] = color
}
return colors
}
I am honestly unable to find any errors after consistent trying, so please help me

Also, Below is my helperFunctions.js file 
tfunction getRandomIntegerBetween(low, high){
try {
if (low<0) throw "Error: Only supports positive integers - low is negative!"
if (low>high) throw "Error: low is greater than high!"
const rangeInclusive = high - low + 1
const RandomValue = Math.floor(Math.random() * rangeInclusive) + low
return RandomValue
}
catch(error) {
throw(`In getRandomIntegerBetween(${low}, ${high}): ${error}`)
}
}
function getParamFromUrl(url, paramId) {
try {
const startParamIdPos = url.lastIndexOf(paramId)
if (startParamIdPos == -1) {
throw new Error(`Parameter: "${paramId}" not present in url: "${url}"`)
}
const startParamValPos = startParamIdPos + paramId.length
const endDelimPos = url.indexOf("&", startParamValPos)
const endParamValPos = (endDelimPos > -1)? endDelimPos: url.length
const param = url.substring( startParamValPos, endParamValPos )
return param
}
catch(error) {
throw("HelperFunctions.js: In getParamFromUrl(url, paramId): " + error)
}
}
I have just copy / pasted the code, but I haven’t understood it yet and it seems also not to be working
So please Help me in figuring it out

In Fact I would suggest if you can spare some time and run my entire code and see where and why these errors are coming ?
I really want to understand and finish these abnormalities, before i move forward on to other courses.
Thanks again

This is my GitHub code:
Please download this zip folder : it contains all my code:
https://github.com/Suveett/CryptoKitties/blob/main/CryptoKitties.zip
Please don’t use the other code, it’s not been updated yet.
Thanks a ton in advance
Suveett kalra
(Su.kal Crypto)