- What can we do with the while statement?
When a while statement is executed, the expression is evaluated with the expression to true(no-zero), the statement executes. But once the statement has finished executing, control returns to the to[ of the while statement and the process is repeated.
- What value needs the statement in the brackets evaluate to in order for the loop to continue?
True statement, once its false, then the while loop will be done.
- What is an infinite loop?
When an expression always evaluates as true, this will make the loop execute forever.
- What is an iteration?
An iteration is when each time a loop executes.
-
When is it a good idea to use a for statement (do a for loop) instead of the while loop we learned previously?
-
How would you write a for-loop in code?
-
What is an off-by-one error?
Quiz
- In the above program, why is variable inner declared inside the while block instead of immediately following the declaration of outer?
This because you want to start counting inward by starting from the smallest variable and then expanding the loop out.
-
Write a program that prints out the letters a through z along with their ASCII codes. Hint: to print characters as integers, you have to use a static_cast.
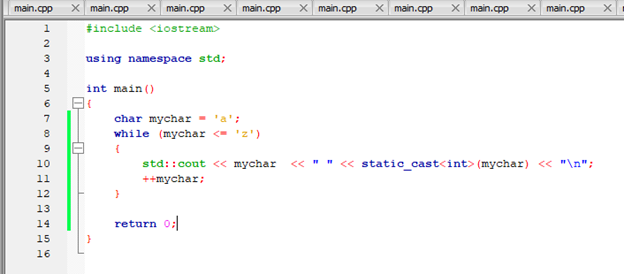
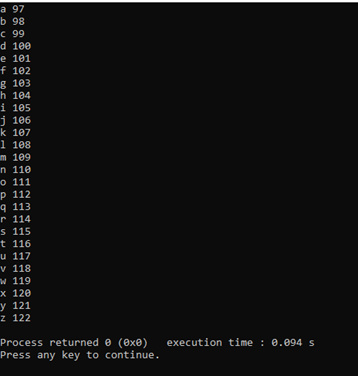
-
Invert the nested loops example so it prints the following:
5 4 3 2 1
4 3 2 1
3 2 1
2 1
1
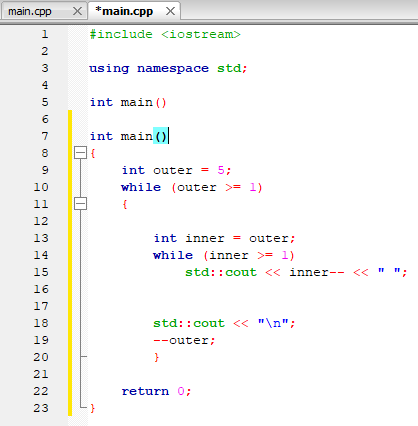
- Now make the numbers print like this:
1
2 1
3 2 1
4 3 2 1
5 4 3 2 1
hint: Figure out how to make it print like this first:
X X X X 1
X X X 2 1
X X 3 2 1
X 4 3 2 1
5 4 3 2 1
- When is it a good idea to use a for statement (do a for loop) instead of the while loop we learned previously?
It is idea when we know exactly how many times we need to iterate, because it lets us easily define, initialize, and change the value of loop variables after each iteration.
- How would you write a for-loop in code?
Its like writing a loop similar to how we write a while loop, but there are additional criteria to consider which are broken down in three parts:
- The init-statement is evaluated. In most cases, the init-statement consists of variable definitions and initialization. This statement is only evaluated once, when the loop is first executed.
- The condition-expression is evaluated. If this evaluates to false, the loop terminates immediately. If this evaluates to true, the statement is executes.
- After the statement is executed, the end-expression is evaluated. For the most part, this expression is used to increment or decrement the variables declared in the init-statement. After the end-expression has been evaluated, the loop returns to the second step mentioned with this answer.
- What is an off-by-one error?
Off-by-one errors occurs when the loop iterates one too many or one too few times. This happens because the wrong relational operator is used in the conditional-expression, like > instead of >=. The errors are hard to detect because the compiler will not error them out, but will continue with the program with the wrong results. The “KEY” here is to remember that loop will execute as long as the conditional-expression is “TRUE”. Plus, in reading the “off-by-one error, it’s a good idea to test your loops to make sure that you get the correct results.
Quiz
-
Write a for loop that prints every even number from 0 to 20.

-
Write a function named sumTo() that takes an integer parameter named value, and returns the sum of all the numbers from 1 to value.
For example, sumTo(5) should return 15, which is 1 + 2 + 3 + 4 + 5.
Hint: Use a non-loop variable to accumulate the sum as you iterate from 1 to the input value, much like the pow() example above uses the total variable to accumulate the return value each iteration.
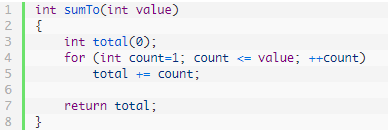
- What’s wrong with the following for loop?
1
2
3 // Print all numbers from 9 to 0
for (unsigned int count=9; count >= 0; --count)
std::cout << count << " ";
This loop will run forever because when the count>=0 it will run until the count is negative but since the count is unsigned will never go negative. The “KEY” here is to not loop on unsigned variables. Although I am still not certain when we will would use an unsigned loop.